Godot How to Hard Edit the Binding for ui_left
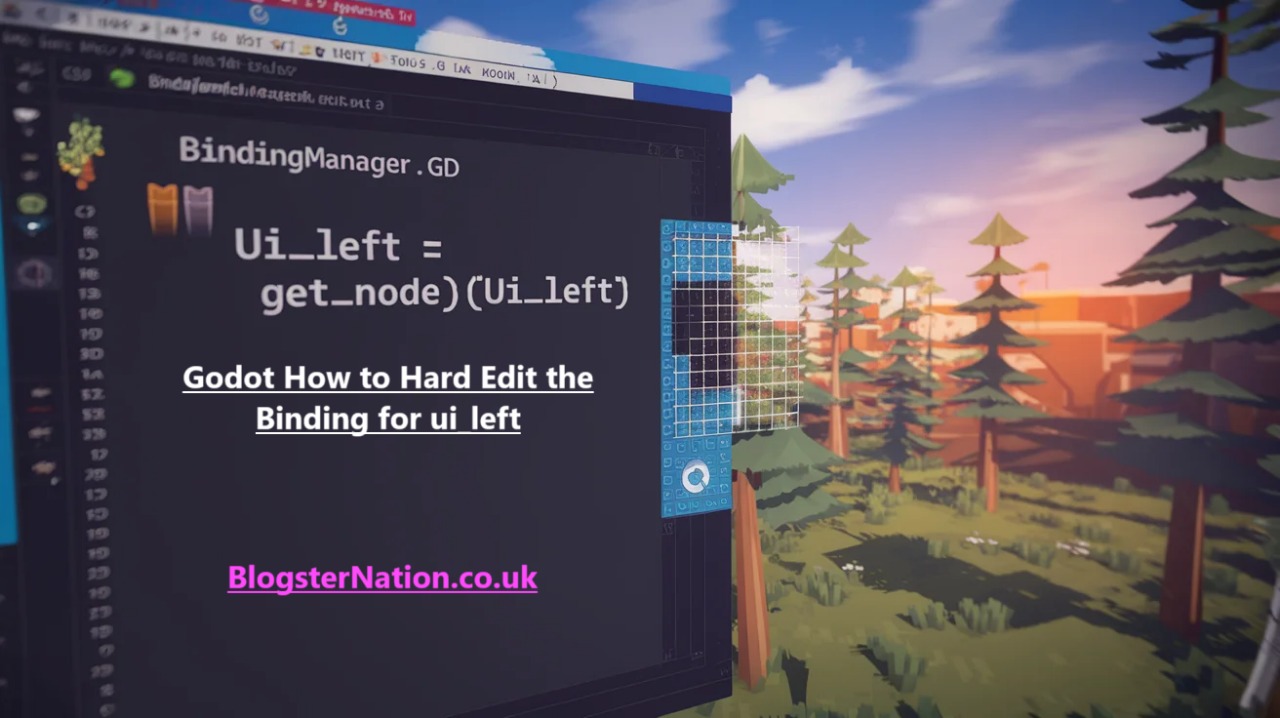
In the Godot engine, input bindings are crucial for customizing player controls, handling UI navigation, and ensuring that the game behaves as expected in response to user input. When working with UI navigation, especially when using keys like ui_left
for player movement or camera control, developers often need to adjust or fine-tune the input bindings for specific actions.
This article will guide you through the process of hard editing the binding for ui_left
in Godot. We will explore how to modify the input actions to achieve custom key bindings and improve the functionality of your project.
Introduction to Input Binding in Godot
In Godot, input actions like ui_left
, ui_right
, ui_up
, and ui_down
are predefined in the engine’s input system, typically used for basic navigation in both UI and 2D/3D space. By default, these bindings map to arrow keys, WASD keys, or other system-specific configurations.
Godot allows you to modify these bindings through its Input Map. You can change the default keys associated with ui_left
or any other action to suit your needs. There are two primary ways to manage bindings:
- Using the Godot Editor’s Input Map settings.
- Hard editing through script for dynamic control or for configurations that need to be programmatically adjusted.
For this article, we’ll focus on the second method: hard editing the binding for ui_left
, using scripts in Godot. This approach is useful when you need to change the control scheme dynamically, or when your game needs to detect and adapt to specific key presses programmatically.
Step-by-Step Process to Hard Edit the Binding for ui_left
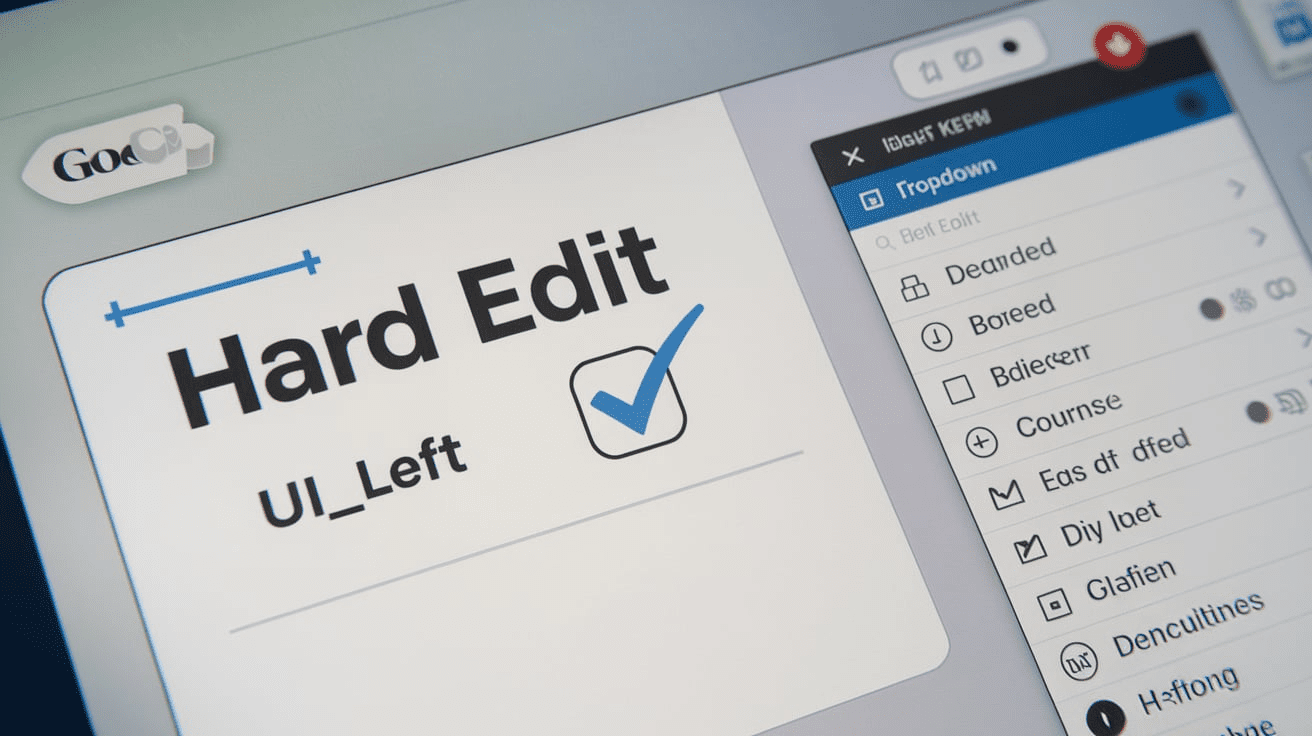
Step 1: Access the Input Map
The first thing you need to do is access the Input Map where the default bindings for various actions like ui_left
are stored.
- Open your Godot project.
- In the top menu, navigate to Project > Project Settings.
- In the Project Settings window, go to the Input Map tab.
- Locate
ui_left
in the list. If it’s not there, you can create it by entering the nameui_left
in the text box and clicking Add.
This is the standard way of configuring bindings, but if you need to programmatically modify it, you’ll have to dive into scripting.
Step 2: Create a Script to Modify ui_left
Binding
To hard edit the binding for ui_left
, you can use a script to map this action to a custom key. Below is a sample code snippet that demonstrates how to do this dynamically:
extends Node
# Function to set a custom key for the 'ui_left' action
func set_custom_ui_left_key():
var new_key = KEY_A # Change this to your desired key (e.g., 'A' key)
# Remove the default binding
InputMap.action_erase_events("ui_left")
# Add the new binding
InputMap.action_add_event("ui_left", InputEventKey.new(new_key))
func _ready():
set_custom_ui_left_key() # Call the function to change the binding
Explanation:
InputMap.action_erase_events("ui_left")
removes any existing input bindings associated withui_left
.InputMap.action_add_event("ui_left", InputEventKey.new(new_key))
adds a new key event binding forui_left
, wherenew_key
can be any key constant likeKEY_A
,KEY_LEFT
, orKEY_RIGHT
.
You can replace KEY_A
with any key you want (e.g., KEY_LEFT
, KEY_KP_LEFT
for arrow keys, or KEY_Q
for a different key). If you need more flexibility in binding, you can use a key from the user’s configuration settings and update it dynamically.
Step 3: Detecting Key Presses for ui_left
Once you have hard-edited the ui_left
action, you can check whether the player has pressed the new key by using Input.is_action_pressed()
or Input.is_action_just_pressed()
:
func _process(delta):
if Input.is_action_pressed("ui_left"):
print("The left key is being pressed!")
This ensures that, regardless of the default configuration, the game responds to your custom key bindings.
Step 4: Allowing Dynamic Changes (Optional)
If you want to let players customize their keybindings in-game (for example, through a settings menu), you can create a system to save and load custom configurations. Use the User Settings or File system to store key mappings, then apply them dynamically as shown in the example.
func save_custom_bindings():
var config = ConfigFile.new()
config.set_value("controls", "ui_left", KEY_A) # Save the key binding for 'ui_left'
config.save("user_settings.cfg")
func load_custom_bindings():
var config = ConfigFile.new()
if config.load("user_settings.cfg") == OK:
var new_key = config.get_value("controls", "ui_left", KEY_LEFT)
InputMap.action_erase_events("ui_left")
InputMap.action_add_event("ui_left", InputEventKey.new(new_key))
Step 5: Testing the New Binding
Once you’ve edited the binding, thoroughly test the input to make sure the new ui_left
key functions as expected. If you’re working on a game with multiple input actions, be sure to check that your changes do not conflict with other key mappings.
Troubleshooting
- Key Not Responding: Ensure that the key event is properly added to the
InputMap
. Also, confirm that the action is being detected in your game logic (e.g., in_process()
or_input()
). - Changes Not Saving: If using custom configurations, check that the file is being saved correctly. You may need to verify file write permissions.
- Conflicting Key Bindings: If you experience issues with multiple bindings for the same action, ensure you are removing old bindings (
action_erase_events()
) before adding new ones.
Conclusion
Hard editing the binding for ui_left
in Godot is a powerful way to fine-tune player input in your game. Whether you’re making custom control schemes for different platforms or allowing players to personalize their controls, the ability to programmatically modify bindings can enhance your game’s flexibility and user experience.
By following the steps outlined above, you can create more dynamic control systems that adapt to different needs. Whether you’re developing a small indie game or a large-scale project, understanding how to work with Godot’s input system can save time and give you the control you need over the user experience.
Frequently Asked Questions (FAQs) About Hard Editing the Binding for ui_left
in Godot:
1. What is the default binding for ui_left
in Godot?
In Godot, the default binding for ui_left
typically maps to the Left Arrow key or A key on the keyboard. This binding is used for movement or navigation in the game’s UI or character controls. The binding can be customized through the Input Map in the Project Settings or modified through script as shown in this article.
2. Can I change the ui_left
binding to any key I want?
Yes, you can change the ui_left
binding to any key by editing the input actions in the Input Map or using GDScript to programmatically assign a new key. For example, you can assign it to the Q key or any other key supported by the engine using the InputMap
API.
3. How can I make the key binding change persistent across game sessions?
To make the key binding changes persistent, you need to save the modified bindings in a configuration file. You can use Godot’s ConfigFile
class to store user preferences (such as key bindings) and load them when the game starts. This allows the player’s custom key settings to persist between sessions.
4. What do I do if the new ui_left
binding doesn’t work in the game?
If your new binding isn’t working, check the following:
- Ensure you are correctly adding the key binding to the Input Map and that it is properly detected in the script.
- Verify that no other bindings conflict with your new key for
ui_left
. - Ensure you are using the correct key code (e.g.,
KEY_A
,KEY_LEFT
) and that it’s recognized by the engine.
5. Can I change other default bindings like ui_up
, ui_right
, and ui_down
using the same method?
Yes, you can apply the same method to modify the bindings for other default actions like ui_up
, ui_right
, and ui_down
. Simply use the corresponding action name (e.g., ui_up
, ui_right
) in the InputMap
and script, and follow the same procedure to remove the old binding and add a new key event.
For More Updates Please Visit Us: Blogsternation.co.uk