Converting Multi-Frame TIFF to GIF in Cross-Platform .NET Environments
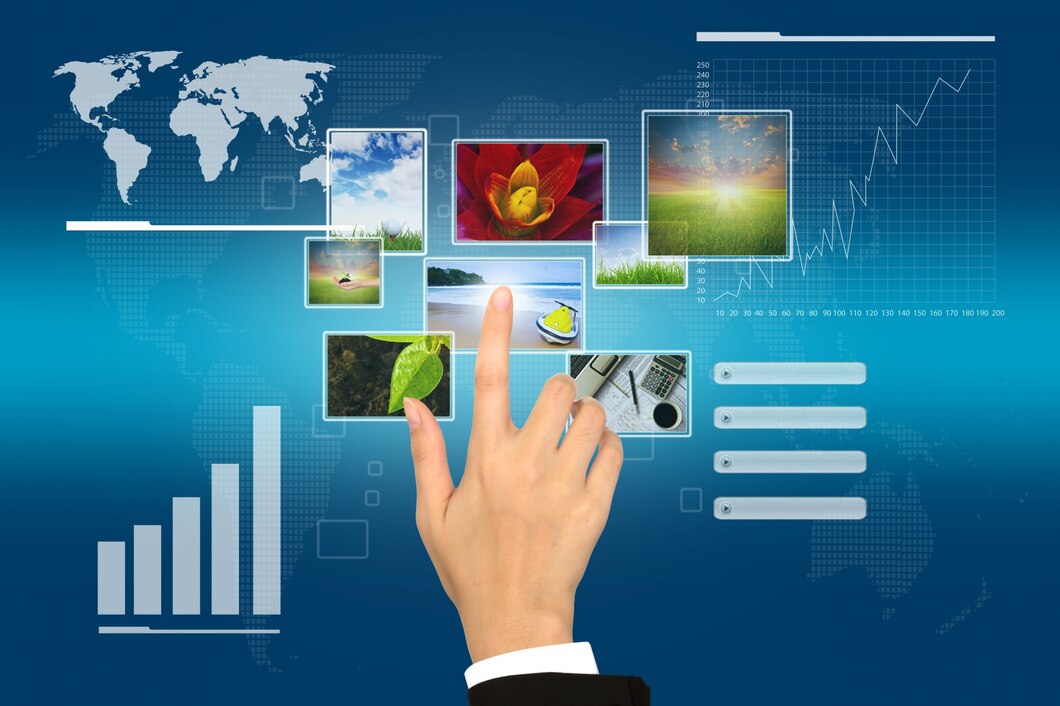
In modern software development, dealing with image files across platforms is an essential task for many developers. One common need is converting multi-frame TIFF (Tagged Image File Format) files into active GIFs in cross-platform .NET environments. TIFF files, often used for storing high-quality, multi-frame images, may need to be converted to GIF format to be displayed as insubstantial cartoons on websites or applications. This article will walk you through the process of converting multi-frame TIFF to GIF, explaining the challenges, tools, and best practices for applying this in cross-platform .NET environments like Windows, Linux, and macOS.
What Are TIFF and GIF?
TIFF (Tagged Image File Format)
It is a useful image format known for packing high-quality images, making it ideal for professional use such as scanning, medical imaging, and document archiving. TIFF files can store multiple images or frames within a single file, which is why it’s often used to save image structures or multi-page documents. While TIFF files provide high image loyalty, they are generally large in size and improper for web usage due to limited browser support.
GIF (Graphics Interchange Format)
GIF, on the other hand, is a widely used image format optimized for animations. GIFs are smaller in file size compared to TIFFs, making them more suitable for online applications. They support multiple frames that can be displayed in sequence, creating simple animations. However, GIFs are limited to 256 colors, which makes them improper for high-color or high-resolution images. Despite these limitations, the small size and cross-platform compatibility of GIFs make them ideal for web use.
The Need for Converting Multi-Frame TIFF to GIF in Cross-Platform .NET Environments
Converting multi-frame TIFF files into GIF format is often necessary when dealing with image sequences that need to be displayed as animations in web applications. In a cross-platform .NET environment, the challenge is compounded by the need to ensure compatibility across Windows, Linux, and macOS.
Traditional .NET libraries such as System.Drawing.Common are platform-dependent and have limited support for non-Windows environments. With .NET 6 and later versions, developers are moving toward cross-platform public library that can handle the conversion efficiently, regardless of the operating system.
Challenges in Converting Multi-Frame TIFF to GIF
1. Platform Compatibility
Many older libraries, such as System.Drawing.Common, are not fully supported across all platforms (especially non-Windows environments like Linux and macOS). This creates a challenge for developers who need to ensure their applications work seamlessly on all operating systems.
2. Managing Multi-Frame TIFFs
TIFF files with multiple frames can be complex to manage. Extracting each frame from a multi-frame TIFF and ensuring that they are correctly resized or adjusted for GIF format can be computationally intensive.
3. Uniform Frame Dimensions
For a GIF to work correctly, all frames must have uniform dimensions. TIFF frames often vary in size or resolution, requiring additional processing to normalise their dimensions before collecting them into a GIF.
Tools and Libraries for Converting Multi-Frame TIFF to GIF
Several cross-platform libraries are available to handle the conversion from TIFF to GIF. Below are some popular choices:
1. ImageSharp
ImageSharp is an open-source, cross-platform library for .NET that excels in image use. It supports a wide range of formats, including TIFF and GIF, and provides an easy-to-use API for image processing tasks. Although ImageSharp does not natively handle multi-frame TIFFs, it can be used in combination with other libraries to process individual frames and generate GIFs.
2. TiffLibrary
TiffLibrary is a specific library designed for handling TIFF files. It offers fine-grained control over TIFF images, making it an ideal choice for interpreting multi-frame TIFFs and processing them into a proper format for GIF conversion.
3. Magick.NET (ImageMagick)
Magick.NET is a powerful .NET cover for the ImageMagick library, offering a rich set of image processing capabilities. It supports multi-frame TIFFs and GIFs, making it an excellent choice for developers needing advanced features such as resizing, color correction, and multi-frame handling.
4. SkiaSharp
SkiaSharp is a cross-platform image processing library that supports a variety of image formats, including TIFF and GIF. While its skills with multi-frame TIFFs are somewhat limited, it can still be used with additional processing steps to handle basic conversion tasks.
Steps to Convert Multi-Frame TIFF to GIF in Cross-Platform .NET Environments
Hereโs a practical approach to convert multi-frame TIFF to GIF using TiffLibrary and ImageSharp:
Step 1: Decode the TIFF File
The first step is to load the multi-frame TIFF file using TiffLibrary. This allows you to access each frame stored within the TIFF file.
using TiffLibrary;
// Open the TIFF file
var tiffImage = Tiff.Load(“path_to_tiff_file.tiff”);
// Iterate over each frame in the TIFF
foreach (var frame in tiffImage.Frames)
{
// Process each frame
}
Step 2: Process Each Frame
Use ImageSharp to resize or adjust the individual frames as needed. Make sure each frame has unchanging dimensions to ensure the GIF functions properly.
using SixLabors.ImageSharp;
using SixLabors.ImageSharp.Processing;
// Resize each frame to match GIF requirements
foreach (var frame in tiffImage.Frames)
{
using (var image = Image.Load(frame.Data))
{
image.Mutate(x => x.Resize(new ResizeOptions
{
Size = new Size(100, 100),
Mode = ResizeMode.Crop
}));
// Process the image further if needed
}
}
Step 3: Assemble the GIF
Once all frames are processed, use ImageSharp to create an animated GIF by combining the frames.
using SixLabors.ImageSharp.Formats.Gif;
// Create an animated GIF with the processed frames
using (var gif = new Image<Rgba32>(frameWidth, frameHeight))
{
gif.Frames.AddFrame(frame);
// Set GIF options (e.g., frame delay, looping)
gif.Save(“output.gif”, new GifEncoder());
}
Best Practices for Converting Multi-Frame TIFF to GIF in Cross-Platform .NET Environments
- Optimize Frame Dimensions: Ensure all frames are resized to consistent dimensions before creating the GIF to avoid issues with uniformity.
- Test Across Platforms: Test the conversion process on Windows, Linux, and macOS to ensure the solution works across all target platforms.
- Handle Transparency: TIFF files may contain transparent areas that need special handling. When converting to GIF, make sure transparency is correctly maintained or converted to a suitable color.
Advantages of Converting Multi-Frame TIFF to GIF in Cross-Platform .NET Environments
- Enhanced Compatibility: Cross-platform libraries like ImageSharp and Magick.NET ensure your solution works effortlessly across Windows, Linux, and macOS.
- Improved Performance: By converting TIFF to GIF, you reduce the file size, making it suitable for web applications and mobile devices.
- Streamlined Animation Creation: Animated GIFs are lightweight and universally supported, making them an ideal format for simple animations.
Conclusion
Converting multi-frame TIFF files to GIFs in cross-platform .NET environments is an essential capability for developers working with dynamic content. By using the right tools, such as ImageSharp, Magick.NET, or TiffLibrary, developers can efficiently manage the conversion process and optimize GIF size and quality. With the right techniques, such as handling transparency and optimizing frame dimensions, you can ensure that the final GIFs work seamlessly across different platforms, offering a smooth user experience.
For More Updates Please Visit Us:ย Blogsternation.co.uk